If the Details Are Wrong It Should Go to Again Enter Details Python

Welcome! If you desire to learn how to work with while loops in Python, then this article is for yous.
While loops are very powerful programming structures that you tin apply in your programs to repeat a sequence of statements.
In this article, you volition learn:
- What while loops are.
- What they are used for.
- When they should be used.
- How they work backside the scenes.
- How to write a while loop in Python.
- What space loops are and how to interrupt them.
- What
while True
is used for and its general syntax. - How to use a
break
statement to stop a while loop.
Yous will learn how while loops work behind the scenes with examples, tables, and diagrams.
Are yous set? Let'south brainstorm. 🔅
🔹 Purpose and Apply Cases for While Loops
Let's start with the purpose of while loops. What are they used for?
They are used to echo a sequence of statements an unknown number of times. This type of loop runs while a given condition is True
and it simply stops when the condition becomes False
.
When we write a while loop, we don't explicitly ascertain how many iterations will be completed, we only write the condition that has to be True
to continue the procedure and Fake
to stop information technology.
💡 Tip: if the while loop status never evaluates to False
, so we volition have an space loop, which is a loop that never stops (in theory) without external intervention.
These are some examples of existent use cases of while loops:
- User Input: When we inquire for user input, nosotros need to check if the value entered is valid. We can't possibly know in accelerate how many times the user will enter an invalid input earlier the program tin continue. Therefore, a while loop would be perfect for this scenario.
- Search: searching for an element in a data structure is some other perfect use case for a while loop because we can't know in accelerate how many iterations will be needed to observe the target value. For example, the Binary Search algorithm tin can exist implemented using a while loop.
- Games: In a game, a while loop could be used to keep the main logic of the game running until the player loses or the game ends. We can't know in advance when this will happen, so this is another perfect scenario for a while loop.
🔸 How While Loops Work
Now that you know what while loops are used for, let's meet their principal logic and how they piece of work behind the scenes. Here we have a diagram:

Let's break this downward in more detail:
- The process starts when a while loop is found during the execution of the programme.
- The condition is evaluated to check if it's
True
orFaux
. - If the condition is
True
, the statements that vest to the loop are executed. - The while loop condition is checked once again.
- If the status evaluates to
True
again, the sequence of statements runs once again and the process is repeated. - When the condition evaluates to
False
, the loop stops and the plan continues across the loop.
One of the most important characteristics of while loops is that the variables used in the loop condition are not updated automatically. We have to update their values explicitly with our code to make sure that the loop will eventually stop when the condition evaluates to False
.
🔹 General Syntax of While Loops
Bang-up. At present you know how while loops work, then let'south dive into the lawmaking and run across how you tin write a while loop in Python. This is the basic syntax:

These are the main elements (in order):
- The
while
keyword (followed by a space). - A condition to decide if the loop will continue running or not based on its truth value (
True
orFalse
). - A colon (
:
) at the end of the first line. - The sequence of statements that will be repeated. This cake of lawmaking is called the "body" of the loop and information technology has to be indented. If a statement is not indented, it will not be considered part of the loop (please see the diagram below).
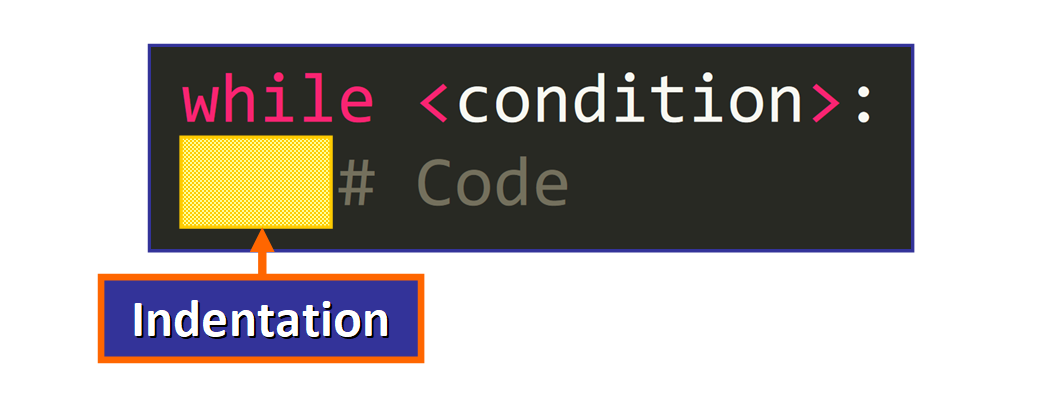
💡 Tip: The Python style guide (PEP eight) recommends using 4 spaces per indentation level. Tabs should only be used to remain consequent with code that is already indented with tabs.
🔸 Examples of While Loops
Now that you know how while loops work and how to write them in Python, let'southward see how they work behind the scenes with some examples.
How a Basic While Loop Works
Here we have a basic while loop that prints the value of i
while i
is less than 8 (i < eight
):
i = 4 while i < 8: print(i) i += 1
If we run the code, we see this output:
4 5 six 7
Let's encounter what happens behind the scenes when the code runs:
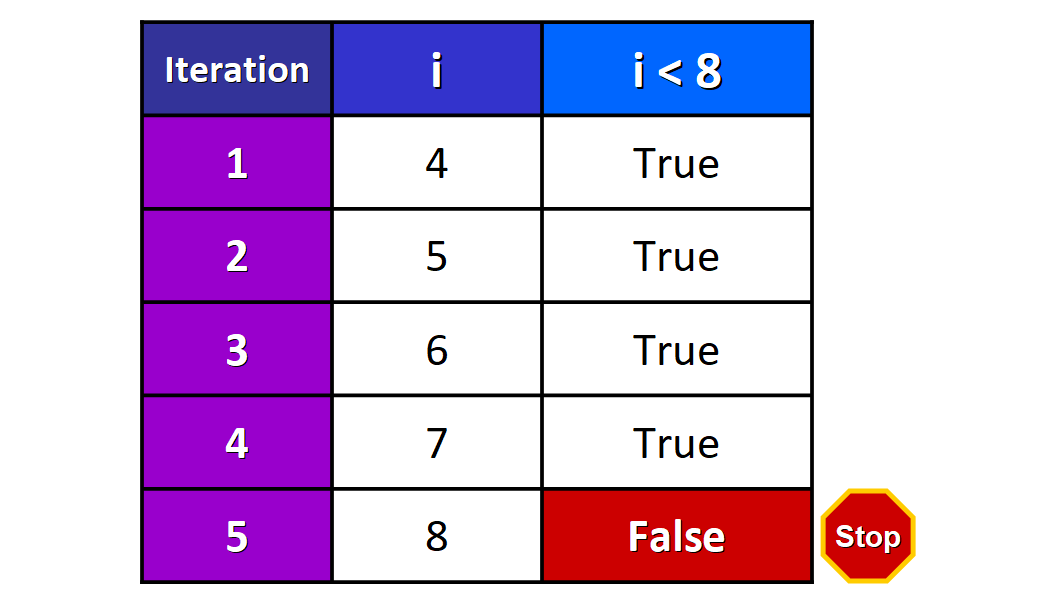
- Iteration ane: initially, the value of
i
is 4, so the conditioni < 8
evaluates toTrue
and the loop starts to run. The value ofi
is printed (iv) and this value is incremented by ane. The loop starts once again. - Iteration two: now the value of
i
is 5, so the statusi < viii
evaluates toTrue
. The torso of the loop runs, the value ofi
is printed (5) and this valuei
is incremented by ane. The loop starts again. - Iterations three and 4: The same procedure is repeated for the third and quaternary iterations, so the integers six and seven are printed.
- Before starting the 5th iteration, the value of
i
is8
. At present the while loop conditioni < 8
evaluates toFaux
and the loop stops immediately.
💡 Tip: If the while loop condition is False
before starting the starting time iteration, the while loop will not fifty-fifty first running.
User Input Using a While Loop
Now allow's see an example of a while loop in a plan that takes user input. We volition the input()
part to ask the user to enter an integer and that integer volition but be appended to list if it'southward even.
This is the code:
# Define the listing nums = [] # The loop will run while the length of the # listing nums is less than 4 while len(nums) < four: # Ask for user input and shop it in a variable as an integer. user_input = int(input("Enter an integer: ")) # If the input is an fifty-fifty number, add it to the list if user_input % 2 == 0: nums.suspend(user_input)
The loop condition is len(nums) < iv
, so the loop will run while the length of the listing nums
is strictly less than four.
Let's clarify this program line by line:
- We starting time by defining an empty list and assigning it to a variable chosen
nums
.
nums = []
- And so, we define a while loop that will run while
len(nums) < 4
.
while len(nums) < four:
- Nosotros inquire for user input with the
input()
function and store it in theuser_input
variable.
user_input = int(input("Enter an integer: "))
💡 Tip: Nosotros demand to convert (cast) the value entered by the user to an integer using the int()
function before assigning it to the variable considering the input()
function returns a string (source).
- We bank check if this value is fifty-fifty or odd.
if user_input % 2 == 0:
- If information technology's even, we append it to the
nums
list.
nums.suspend(user_input)
- Else, if information technology'southward odd, the loop starts once again and the condition is checked to determine if the loop should continue or not.
If we run this code with custom user input, we get the post-obit output:
Enter an integer: 3 Enter an integer: 4 Enter an integer: ii Enter an integer: 1 Enter an integer: 7 Enter an integer: six Enter an integer: 3 Enter an integer: iv
This table summarizes what happens behind the scenes when the code runs:

💡 Tip: The initial value of len(nums)
is 0
because the list is initially empty. The concluding column of the tabular array shows the length of the list at the end of the current iteration. This value is used to check the condition before the next iteration starts.
Equally you lot can see in the table, the user enters fifty-fifty integers in the second, third, sixth, and eight iterations and these values are appended to the nums
list.
Earlier a "ninth" iteration starts, the status is checked over again merely now it evaluates to False
because the nums
list has four elements (length 4), and so the loop stops.
If we check the value of the nums
listing when the procedure has been completed, we see this:
>>> nums [four, 2, 6, iv]
Exactly what nosotros expected, the while loop stopped when the condition len(nums) < 4
evaluated to False
.
Now you know how while loops work behind the scenes and you've seen some practical examples, and then allow'southward dive into a key chemical element of while loops: the status.
🔹 Tips for the Condition in While Loops
Earlier y'all get-go working with while loops, you should know that the loop condition plays a fundamental role in the functionality and output of a while loop.

Y'all must exist very careful with the comparison operator that you choose because this is a very mutual source of bugs.
For example, common errors include:
- Using
<
(less than) instead of<=
(less than or equal to) (or vice versa). - Using
>
(greater than) instead of>=
(greater than or equal to) (or vice versa).
This can bear upon the number of iterations of the loop and even its output.
Let's see an example:
If we write this while loop with the condition i < 9
:
i = 6 while i < ix: print(i) i += 1
We run into this output when the code runs:
6 seven 8
The loop completes iii iterations and it stops when i
is equal to nine
.
This tabular array illustrates what happens behind the scenes when the code runs:
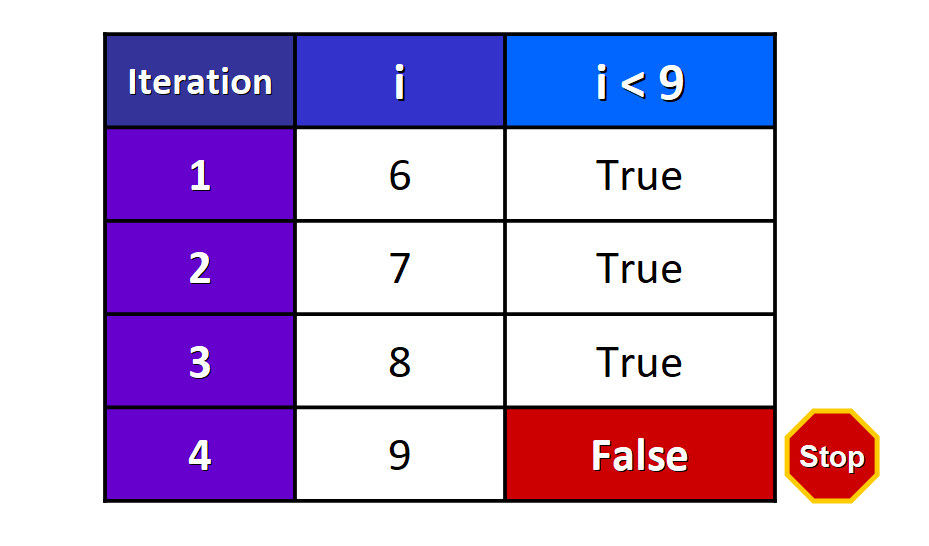
- Before the starting time iteration of the loop, the value of
i
is six, so the statusi < nine
isTrue
and the loop starts running. The value ofi
is printed and then information technology is incremented by i. - In the second iteration of the loop, the value of
i
is 7, so the statusi < ix
isTrue
. The body of the loop runs, the value ofi
is printed, then it is incremented past one. - In the third iteration of the loop, the value of
i
is 8, and then the conditioni < 9
isTruthful
. The torso of the loop runs, the value ofi
is printed, and then it is incremented by ane. - The condition is checked once more before a quaternary iteration starts, just now the value of
i
is 9, soi < nine
isFalse
and the loop stops.
In this case, we used <
as the comparing operator in the condition, just what do you call up volition happen if we employ <=
instead?
i = half-dozen while i <= 9: print(i) i += 1
We see this output:
6 7 viii 9
The loop completes one more iteration because at present nosotros are using the "less than or equal to" operator <=
, so the condition is still Truthful
when i
is equal to ix
.
This table illustrates what happens behind the scenes:

Four iterations are completed. The status is checked over again earlier starting a "fifth" iteration. At this point, the value of i
is 10
, so the condition i <= 9
is Simulated
and the loop stops.
🔸 Space While Loops
Now you know how while loops piece of work, but what practice y'all think will happen if the while loop condition never evaluates to False
?
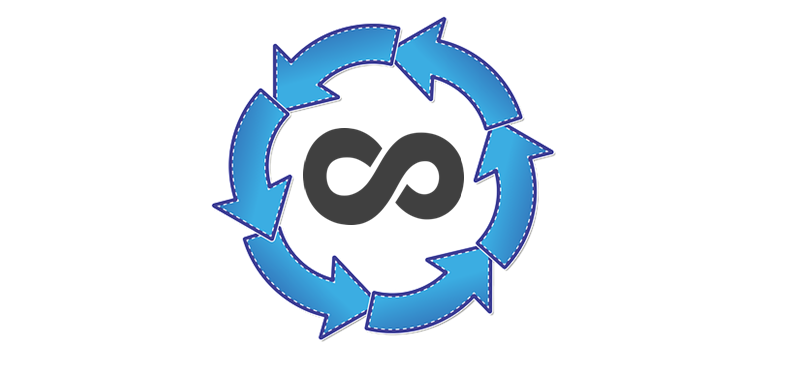
What are Infinite While Loops?
Recollect that while loops don't update variables automatically (we are in accuse of doing that explicitly with our code). Then there is no guarantee that the loop will stop unless we write the necessary code to brand the status False
at some point during the execution of the loop.
If we don't do this and the status always evaluates to True
, then we volition have an infinite loop, which is a while loop that runs indefinitely (in theory).
Infinite loops are typically the event of a bug, just they can also exist caused intentionally when we want to echo a sequence of statements indefinitely until a pause
statement is found.
Let's see these two types of infinite loops in the examples below.
💡 Tip: A bug is an error in the program that causes incorrect or unexpected results.
Example of Infinite Loop
This is an example of an unintentional infinite loop caused by a issues in the programme:
# Define a variable i = 5 # Run this loop while i is less than 15 while i < xv: # Print a message print("Hi, World!")
Analyze this code for a moment.
Don't you notice something missing in the trunk of the loop?
That'south right!
The value of the variable i
is never updated (information technology's always v
). Therefore, the condition i < fifteen
is always Truthful
and the loop never stops.
If we run this code, the output volition be an "space" sequence of Hi, World!
messages because the body of the loop print("Howdy, Globe!")
volition run indefinitely.
Hi, World! How-do-you-do, World! Hello, World! Hullo, Earth! Hello, Earth! Howdy, World! Hello, Globe! Hello, World! How-do-you-do, World! Hello, Earth! Howdy, World! Hello, World! Hello, World! Hello, World! Hello, Earth! Howdy, Globe! Howdy, Globe! Hello, Globe! . . . # Continues indefinitely
To stop the program, nosotros will need to interrupt the loop manually past pressing CTRL + C
.
When we practise, nosotros will encounter a KeyboardInterrupt
error similar to this ane:

To prepare this loop, we volition need to update the value of i
in the torso of the loop to make sure that the condition i < 15
will eventually evaluate to Faux
.
This is i possible solution, incrementing the value of i
by 2 on every iteration:
i = 5 while i < 15: print("Hello, World!") # Update the value of i i += 2
Groovy. At present y'all know how to set up infinite loops caused by a problems. Y'all just need to write code to guarantee that the condition volition somewhen evaluate to Fake
.
Let's first diving into intentional infinite loops and how they work.
🔹 How to Brand an Infinite Loop with While Truthful
We tin generate an space loop intentionally using while Truthful
. In this example, the loop will run indefinitely until the procedure is stopped by external intervention (CTRL + C
) or when a break
statement is found (you lot volition larn more about break
in but a moment).
This is the basic syntax:

Instead of writing a status after the while
keyword, we just write the truth value directly to betoken that the condition volition always be True
.
Hither we have an case:
>>> while True: print(0) 0 0 0 0 0 0 0 0 0 0 0 0 0 Traceback (almost recent call last): File "<pyshell#ii>", line 2, in <module> print(0) KeyboardInterrupt
The loop runs until CTRL + C
is pressed, but Python also has a interruption
statement that we can use straight in our code to finish this type of loop.
The interruption
statement
This argument is used to stop a loop immediately. Y'all should retrieve of information technology as a red "finish sign" that you lot tin can utilize in your code to accept more control over the behavior of the loop.

Co-ordinate to the Python Documentation:
Theinterruption
statement, similar in C, breaks out of the innermost enclosingfor
orwhile
loop.
This diagram illustrates the bones logic of the break
statement:

break
statement This is the bones logic of the suspension
statement:
- The while loop starts only if the condition evaluates to
Truthful
. - If a
intermission
statement is found at any indicate during the execution of the loop, the loop stops immediately. - Else, if
break
is non plant, the loop continues its normal execution and it stops when the condition evaluates toFalse
.
We tin employ break
to stop a while loop when a condition is met at a item point of its execution, so y'all will typically find it within a conditional statement, similar this:
while True: # Lawmaking if <condition>: break # Lawmaking
This stops the loop immediately if the condition is True
.
💡 Tip: You can (in theory) write a break
statement anywhere in the body of the loop. Information technology doesn't necessarily have to be part of a conditional, but we ordinarily use it to finish the loop when a given condition is True
.
Here we have an example of break
in a while Truthful
loop:
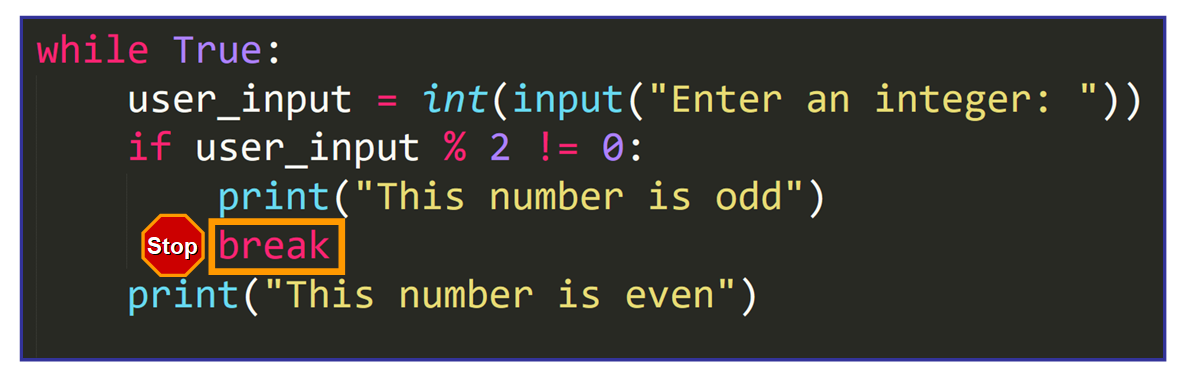
Let's meet information technology in more particular:
The first line defines a while True
loop that will run indefinitely until a break
statement is found (or until it is interrupted with CTRL + C
).
while Truthful:
The second line asks for user input. This input is converted to an integer and assigned to the variable user_input
.
user_input = int(input("Enter an integer: "))
The tertiary line checks if the input is odd.
if user_input % 2 != 0:
If information technology is, the message This number is odd
is printed and the break
argument stops the loop immediately.
impress("This number of odd") break
Else, if the input is fifty-fifty , the message This number is even
is printed and the loop starts once again.
print("This number is even")
The loop will run indefinitely until an odd integer is entered because that is the simply way in which the break
statement will exist found.
Here we have an case with custom user input:
Enter an integer: 4 This number is even Enter an integer: half dozen This number is even Enter an integer: eight This number is fifty-fifty Enter an integer: three This number is odd >>>
🔸 In Summary
- While loops are programming structures used to repeat a sequence of statements while a condition is
True
. They terminate when the condition evaluates toImitation
. - When yous write a while loop, you need to make the necessary updates in your code to brand sure that the loop will eventually cease.
- An infinite loop is a loop that runs indefinitely and it only stops with external intervention or when a
pause
statement is establish. - You tin can finish an space loop with
CTRL + C
. - You can generate an infinite loop intentionally with
while True
. - The
break
statement can be used to stop a while loop immediately.
I really hope you liked my article and institute it helpful. Now you lot know how to piece of work with While Loops in Python.
Follow me on Twitter @EstefaniaCassN and if you lot want to learn more than nearly this topic, check out my online course Python Loops and Looping Techniques: Beginner to Advanced.
Learn to lawmaking for gratuitous. freeCodeCamp'south open source curriculum has helped more than 40,000 people go jobs every bit developers. Get started
Source: https://www.freecodecamp.org/news/python-while-loop-tutorial/
0 Response to "If the Details Are Wrong It Should Go to Again Enter Details Python"
Postar um comentário